For now flex is not very popular in maven community and there are not so many repositories for flex libraries and plugins as well. So I'd like to recommend a great plugin for maven to compile flex applications - Flexmojos from Sonatype. It's well documented, supporting flex 2-4 versions, rsl, modules, localization and has it's own repositories.
For those who haven't work with maven at all, I strongly recommend to read this book at least first two chapters to understand the philosophy of maven. For those who think that he or she is a God of maven then I recommend to read the same book from chapter 17.
As for me I like to use maven as much as possible. So first I'll create a folder structure for our flex project. For this action maven has a great plugin archetype that will create a folder structure and necessary pom.xml files for you.
Flexmojos has its own archetypes for flex application, so here they are:
Create library:
mvn archetype:generate -DarchetypeRepository=http://repository.sonatype.org/content/groups/public -DarchetypeGroupId=org.sonatype.flexmojos -DarchetypeArtifactId=flexmojos-archetypes-library -DarchetypeVersion=3.2.0
Create application:
mvn archetype:generate -DarchetypeRepository=http://repository.sonatype.com/content/groups/public -DarchetypeGroupId=org.sonatype.flexmojos -DarchetypeArtifactId=flexmojos-archetypes-application -DarchetypeVersion=3.2.0
Create modular application:
mvn archetype:generate -DarchetypeRepository=http://repository.sonatype.org/content/groups/public -DarchetypeGroupId=org.sonatype.flexmojos -DarchetypeArtifactId=flexmojos-archetypes-modular-webapp -DarchetypeVersion=3.2.0
We will use the second one flexmojos-archetype-application to create a simple flex application. To run this archetype simply copy it from post and past into a shell. You'll need to answer some questions before the plugin will create you a folder structure. If everything was good you'll be able to have such a screen.
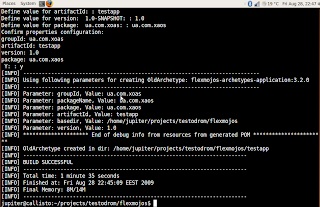
Now let's take a look at the folder structure that maven has created for us.
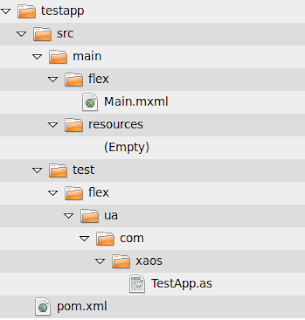
But the most interesting part is pom.xml. Let's take a look.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>ua.com.xoas</groupId> <artifactId>testapp</artifactId> <version>1.0</version> <packaging>swf</packaging> <name>testapp Flex</name> <build> <sourceDirectory>src/main/flex</sourceDirectory> <testSourceDirectory>src/test/flex</testSourceDirectory> <plugins> <plugin> <groupId>org.sonatype.flexmojos</groupId> <artifactId>flexmojos-maven-plugin</artifactId> <version>3.2.0</version> <extensions>true</extensions> </plugin> </plugins> </build> <dependencies> <dependency> <groupId>com.adobe.flex.framework</groupId> <artifactId>flex-framework</artifactId> <version>3.2.0.3958</version> <type>pom</type> </dependency> <dependency> <groupId>org.sonatype.flexmojos</groupId> <artifactId>flexmojos-unittest-support</artifactId> <version>3.2.0</version> <type>swc</type> <scope>test</scope> </dependency> </dependencies> </project>
If you need to configure your build simply add a configuration element inside of the build element, like this:
<build> <sourceDirectory>src/main/flex</sourceDirectory> <testSourceDirectory>src/test/flex</testSourceDirectory> <plugins> <plugin> <groupId>org.sonatype.flexmojos</groupId> <artifactId>flexmojos-maven-plugin</artifactId> <version>3.2.0</version> <extensions>true</extensions> <configuration> <!-- put your configuration options here --> <debug>true</debug> <allowSourcePathOverlap>true</allowSourcePathOverlap> </configuration> </plugin> </plugins> </build>
I've added two more options to build:
debug - Turn on generation of debuggable SWFs.
allowSourcePathOverlap - Allow the source-path to have path-elements which contain other path-elements.
Use this link to get a list of available configuration options.
To compile this application you'll need to run: mvn install -DflashPlayer.command={path to flexplayer} (path to flashplayer is required otherwise you'll get an error during test phase) If you want to skip test phase run this: mvn install -Dmaven.test.skip=true
The last thing I'd like to pay your attention to is dependencies element. One of the pros of the Flexmojos is that you don't have to install flex-sdk - it will be downloaded automatically and all you need is to add a dependency, in "maven style", to flex-sdk.
<dependency> <groupId>com.adobe.flex.framework</groupId> <artifactId>flex-framework</artifactId> <version>3.2.0.3958</version> <type>pom</type> </dependency>P.S.
Great documentation, great support makes Flexmojos the best plugin for maven to compile flex application.
Almost forgot: use flexmojos:flexbuilder goal to configure environment files for flexbuilder.
Have a nice code :)